mirror of
https://github.com/NGSolve/netgen.git
synced 2024-11-12 00:59:16 +05:00
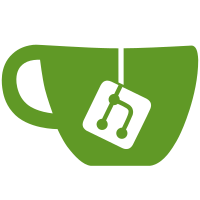
- Replace PYBIND11_PLUGIN with PYBIND11_MODULE - Fix warnings about symbol visibility by replacing 'namespace pybind11' with 'namespace PYBIND11_NAMESPACE' - Pybind sets the default visibility of its namespace to 'hidden' Thus, our export functions like ExportCSG(py::module &m) also are hidden by default. To work around that define DLL_HEADER '__attribute__ ((visibility ("default"))) on GNUC platforms.
72 lines
1.4 KiB
C++
72 lines
1.4 KiB
C++
#ifdef NG_PYTHON
|
|
|
|
#include <pybind11/pybind11.h>
|
|
#include <pybind11/operators.h>
|
|
namespace py = pybind11;
|
|
#include <iostream>
|
|
#include <sstream>
|
|
|
|
|
|
namespace PYBIND11_NAMESPACE {
|
|
template<typename T>
|
|
bool CheckCast( py::handle obj ) {
|
|
try{
|
|
obj.cast<T>();
|
|
return true;
|
|
}
|
|
catch (py::cast_error &e) {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
|
|
template <typename T>
|
|
struct extract
|
|
{
|
|
py::handle obj;
|
|
extract( py::handle aobj ) : obj(aobj) {}
|
|
|
|
bool check() { return CheckCast<T>(obj); }
|
|
T operator()() { return obj.cast<T>(); }
|
|
};
|
|
}
|
|
|
|
struct NGDummyArgument {};
|
|
|
|
inline void NOOP_Deleter(void *) { ; }
|
|
|
|
namespace netgen
|
|
{
|
|
|
|
//////////////////////////////////////////////////////////////////////
|
|
// Lambda to function pointer conversion
|
|
template <typename Function>
|
|
struct function_traits
|
|
: public function_traits<decltype(&Function::operator())> {};
|
|
|
|
template <typename ClassType, typename ReturnType, typename... Args>
|
|
struct function_traits<ReturnType(ClassType::*)(Args...) const> {
|
|
typedef ReturnType (*pointer)(Args...);
|
|
typedef ReturnType return_type;
|
|
};
|
|
|
|
template <typename Function>
|
|
typename function_traits<Function>::pointer
|
|
FunctionPointer (const Function& lambda) {
|
|
return static_cast<typename function_traits<Function>::pointer>(lambda);
|
|
}
|
|
|
|
|
|
template <class T>
|
|
inline std::string ToString (const T& t)
|
|
{
|
|
std::stringstream ss;
|
|
ss << t;
|
|
return ss.str();
|
|
}
|
|
|
|
}
|
|
|
|
#endif
|
|
|